FEM.Elements.E2D package#
Collection of 2D Elements
Submodules#
FEM.Elements.E2D.Element2D module#
Defines a general 2D element
- class FEM.Elements.E2D.Element2D.Element2D(coords: numpy.ndarray, _coords: numpy.ndarray, gdl: numpy.ndarray, **kargs)#
Bases:
FEM.Elements.Element.Element
Create a 2D element
- Parameters
coords (np.ndarray) – Element coordinate matrix
_coords (np.ndarray) – Element coordinate matrix for graphical interface purposes
gdl (np.ndarray) – Degree of freedom matrix
- draw() None #
Create a graph of element
- isInside(x: numpy.ndarray) numpy.ndarray #
Test if a given points is inside element domain
- Parameters
x (np.ndarray) – Point to be tested
- Returns
Bolean array of test result
- Return type
np.ndarray
- jacobianGraph() None #
Create the determinant jacobian graph
FEM.Elements.E2D.LTriangular module#
TRIANGULAR ELEMENT#
Defines the lagrange first order triangular element
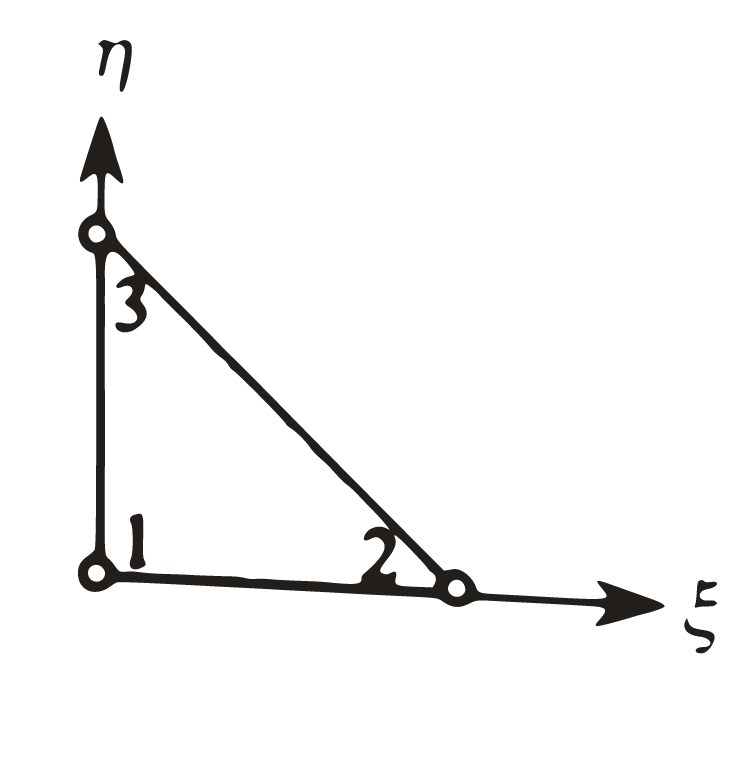
3 nodes triangular element. (Reddy, 2005)#
Shape Functions#
Shape Functions Derivatives#
- class FEM.Elements.E2D.LTriangular.LTriangular(coords: numpy.ndarray, gdl: numpy.ndarray, n: int = 2, **kargs)#
Bases:
FEM.Elements.E2D.Element2D.Element2D
,FEM.Elements.E2D.TriangularScheme.TriangularScheme
Creates a lagrangian triangular element of order 1
- Parameters
coords (np.ndarray) – Element coordinates matrix
gdl (np.ndarray) – Element gdl matrix
n (int, optional) – Number of Gauss Points. Defaults to 2.
- dpsis(z: numpy.ndarray) numpy.ndarray #
Calculates the shape functions derivatives of a given natural coordinates
- Parameters
z (np.ndarray) – Natural coordinates matrix
- Returns
Shape function derivatives evaluated in Z points
- Return type
np.ndarray
- psis(z: numpy.ndarray) numpy.ndarray #
Calculates the shape functions of a given natural coordinates
- Parameters
z (np.ndarray) – Natural coordinates matrix
- Returns
Shape function evaluated in Z points
- Return type
np.ndarray
FEM.Elements.E2D.QTriangular module#
TRIANGULAR SECOND ORDER ELEMENT#
Defines the lagrange second order triangular element
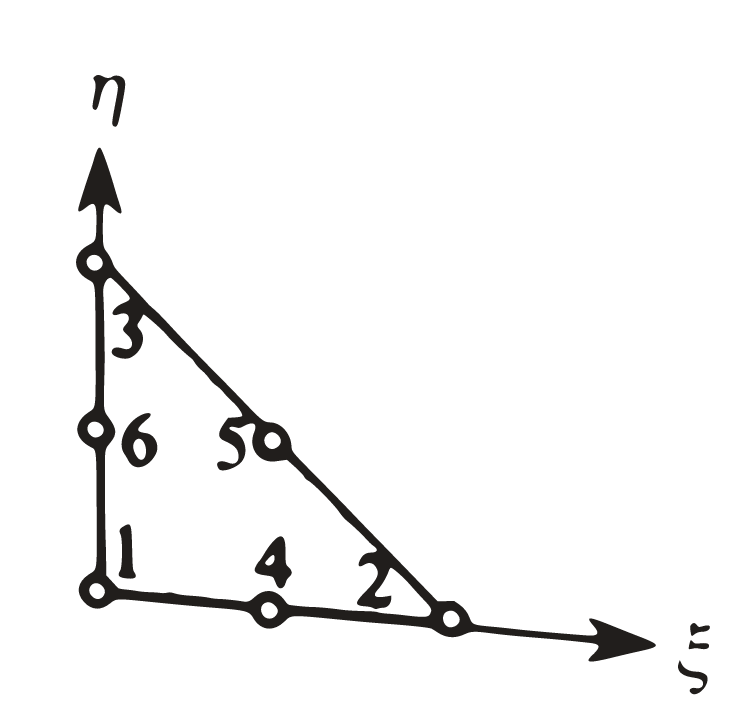
6 nodes triangular element. (Reddy, 2005)#
Shape Functions#
Shape Functions Derivatives#
- class FEM.Elements.E2D.QTriangular.QTriangular(coords: numpy.ndarray, gdl: numpy.ndarray, n: int = 3, **kargs)#
Bases:
FEM.Elements.E2D.Element2D.Element2D
,FEM.Elements.E2D.TriangularScheme.TriangularScheme
Creates a lagrangian element of order 2
- Parameters
coords (np.ndarray) – Element coordinates matrix
gdl (np.ndarray) – Element gdl matrix
n (int, optional) – Number of Gauss Points. Defaults to 2.
- dpsis(z: numpy.ndarray) numpy.ndarray #
Calculates the shape functions derivatives of a given natural coordinates
- Parameters
z (np.ndarray) – Natural coordinates matrix
- Returns
Shape function derivatives evaluated in Z points
- Return type
np.ndarray
- psis(z: numpy.ndarray) numpy.ndarray #
Calculates the shape functions of a given natural coordinates
- Parameters
z (np.ndarray) – Natural coordinates matrix
- Returns
Shape function evaluated in Z points
- Return type
np.ndarray
FEM.Elements.E2D.Quadrilateral module#
QUADRILATERAL ELEMENT#
Defines the lagrange first order quadrilateral element
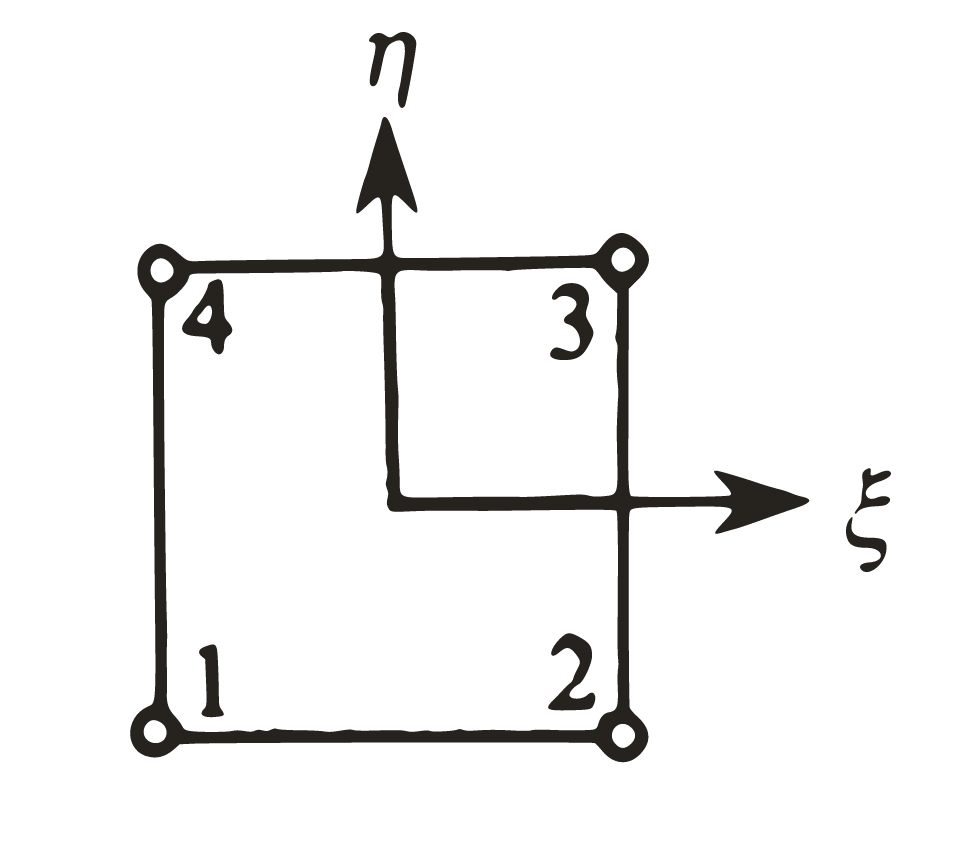
4 nodes quadrilateral element. (Reddy, 2005)#
Shape Functions#
Shape Functions Derivatives#
- class FEM.Elements.E2D.Quadrilateral.Quadrilateral(coords: numpy.ndarray, gdl: numpy.ndarray, n: int = 2, **kargs)#
Bases:
FEM.Elements.E2D.Element2D.Element2D
,FEM.Elements.E2D.RectangularScheme.RectangularScheme
Creates a lagrangian rectangular element of order 1
- Parameters
coords (np.ndarray) – Element coordinates matrix
gdl (np.ndarray) – Element gdl matrix
n (int, optional) – Number of Gauss Points. Defaults to 2.
- dpsis(z: numpy.ndarray) numpy.ndarray #
Calculates the shape functions derivatives of a given natural coordinates
- Parameters
z (np.ndarray) – Natural coordinates matrix
- Returns
Shape function derivatives evaluated in Z points
- Return type
np.ndarray
- psis(z: numpy.ndarray) numpy.ndarray #
Calculates the shape functions of a given natural coordinates
- Parameters
z (np.ndarray) – Natural coordinates matrix
- Returns
Shape function evaluated in Z points
- Return type
np.ndarray
FEM.Elements.E2D.RectangularScheme module#
Define the rectangular scheme used by rectangular elements
- class FEM.Elements.E2D.RectangularScheme.RectangularScheme(n: int, **kargs)#
Bases:
object
Generates a rectangular integration scheme
- Parameters
n (int) – Number of gauss points
FEM.Elements.E2D.Serendipity module#
QUADRILATERAL SERENDIPITY ELEMENT#
Defines the serendipity second order quadrilateral element
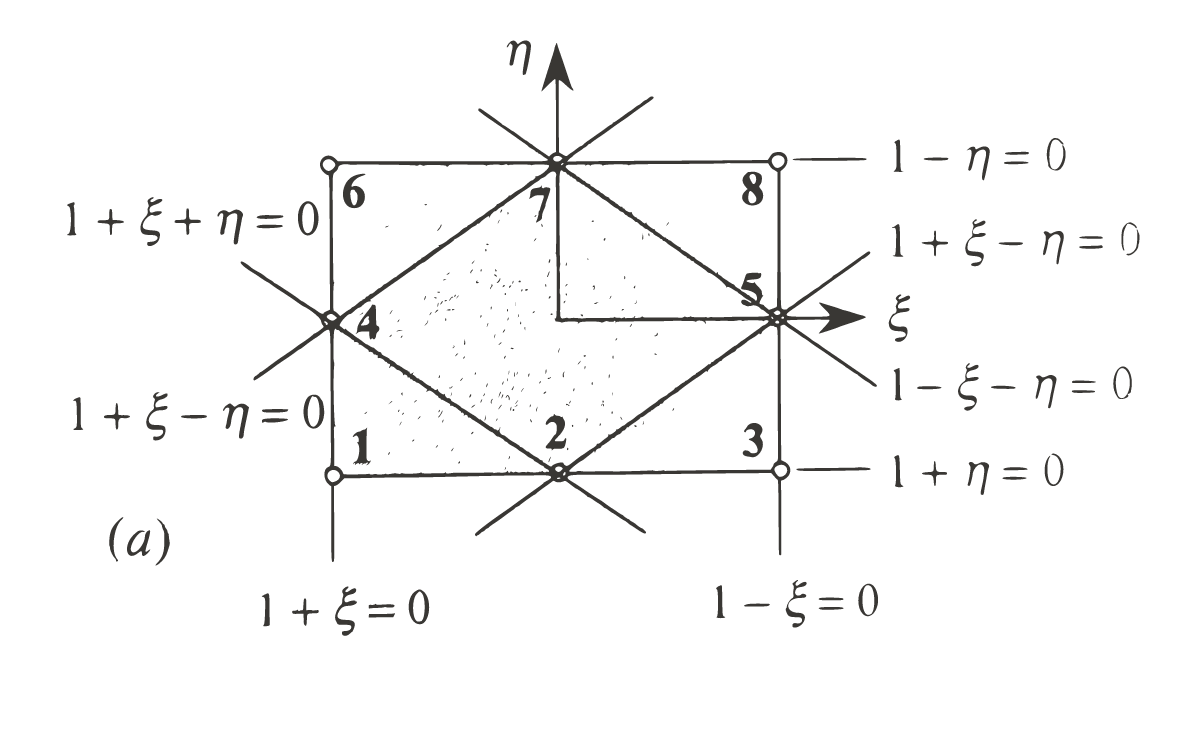
8 nodes quadrilateral element. (Reddy, 2005)#
Shape Functions#
Shape Functions Derivatives#
- class FEM.Elements.E2D.Serendipity.Serendipity(coords: numpy.ndarray, gdl: numpy.ndarray, n: int = 3, **kargs)#
Bases:
FEM.Elements.E2D.Element2D.Element2D
,FEM.Elements.E2D.RectangularScheme.RectangularScheme
Creates a Serendipity element
- Parameters
coords (np.ndarray) – Coordinate matrix of element
gdl (np.ndarray) – Coordinate matrix of element GDL’s
n (int, optional) – Number of gauss points. Defaults to 3.
- dpsis(z: numpy.ndarray) numpy.ndarray #
Calculates the shape functions derivatives of a given natural coordinates
- Parameters
z (np.ndarray) – Natural coordinates matrix
- Returns
Shape function derivatives evaluated in Z points
- Return type
np.ndarray
- psis(z: numpy.ndarray) numpy.ndarray #
Calculates the shape functions of a given natural coordinates
- Parameters
z (np.ndarray) – Natural coordinates matrix
- Returns
Shape function evaluated in Z points
- Return type
np.ndarray
FEM.Elements.E2D.TriangularScheme module#
Define the triangular scheme used by triangular elements
- class FEM.Elements.E2D.TriangularScheme.TriangularScheme(n: int, **kargs)#
Bases:
object
Generate a triangular integration scheme
- Parameters
n (int) – Number of gauss points